The giants behind javascript/web.
Render Engine
- Webkit: Opened source by Apple, web render engine for Safari, IOS, Gnome
- Blink: Core of Chrome, forked branch from Webkit by Google
- Webkit2GTK: Platform to build native UI with HTML/CSS. Render engine of Gnome Desktop.
- Gecko: Firefox browser and Thunderbird email client
- KHTML: Developed by KDE community, origin of Webkit, EdgeHTML
Webkit Architecture
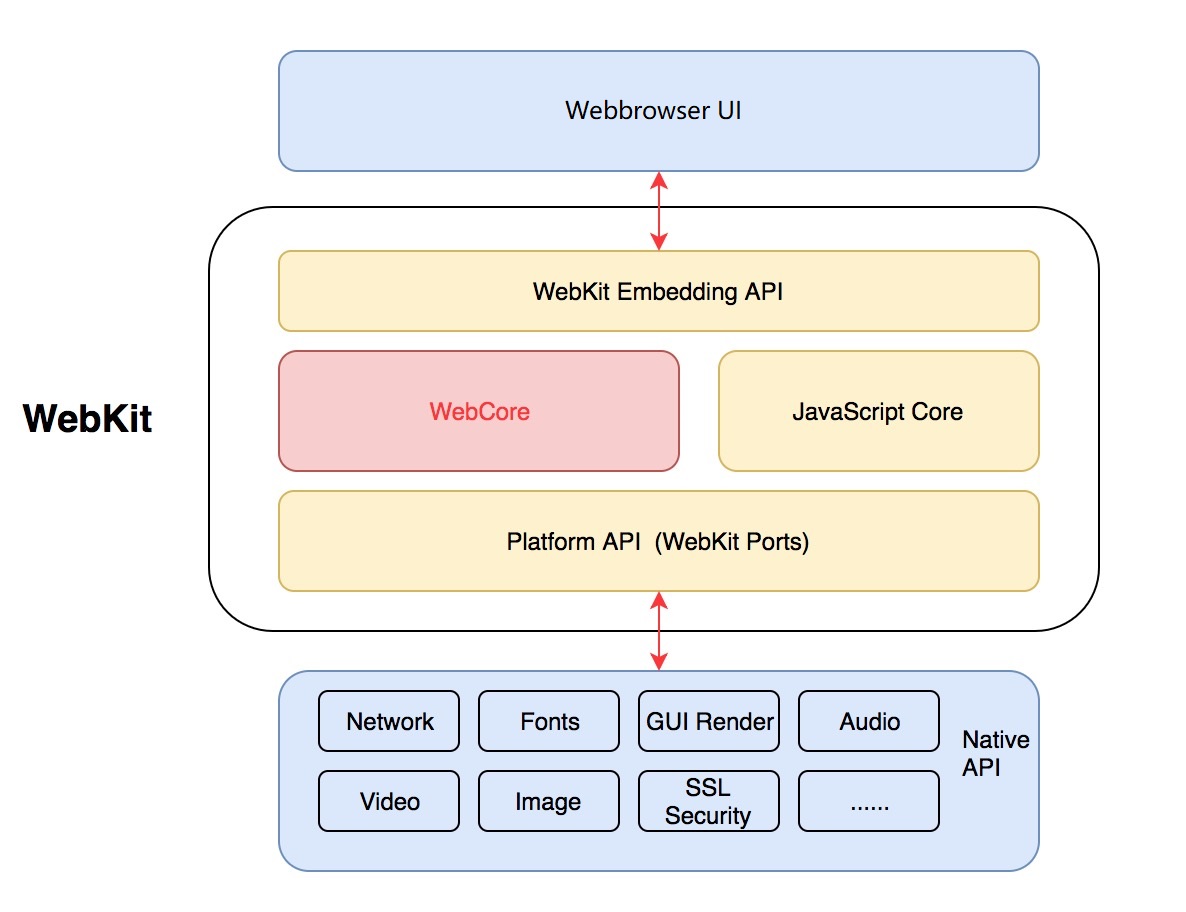
- Webkit Embedding API is for UI interaction between webbrowser UI & webkit.
- Webkit Ports is abstract layer interacted with different OS/platform.
WebCore
WebCore implementation impacts HTML compatibility.
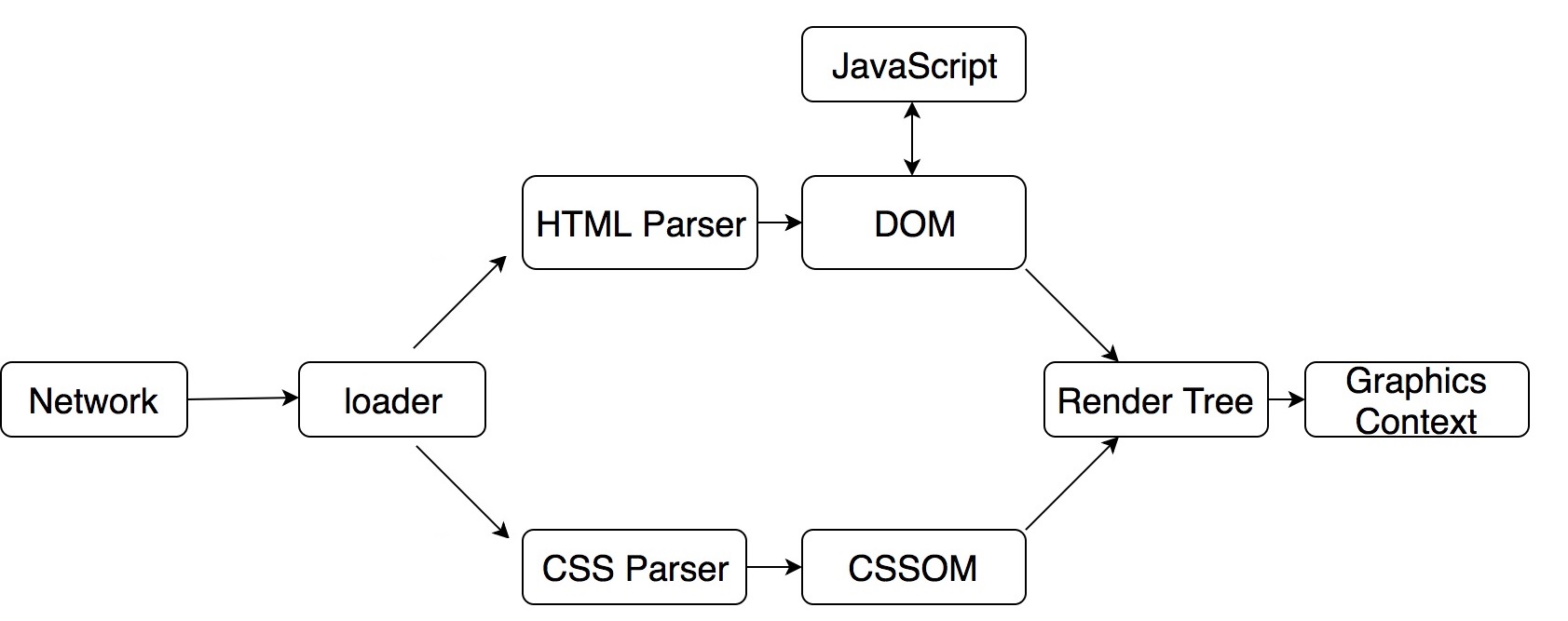
JavascriptCore
Javascript engine which interpreters and executes javascript.
Roughly Javascript Engine = Interpreter + JIT Compiler + GC
- JavascriptCore by Apple, written in C++, system level framework of IOS
- V8 by google, written in C++, best of performance, also powers Node.js
- SpiderMonkey by Mozilla, the very first javascript engine, still powers Firefox today, written in C++/Rust
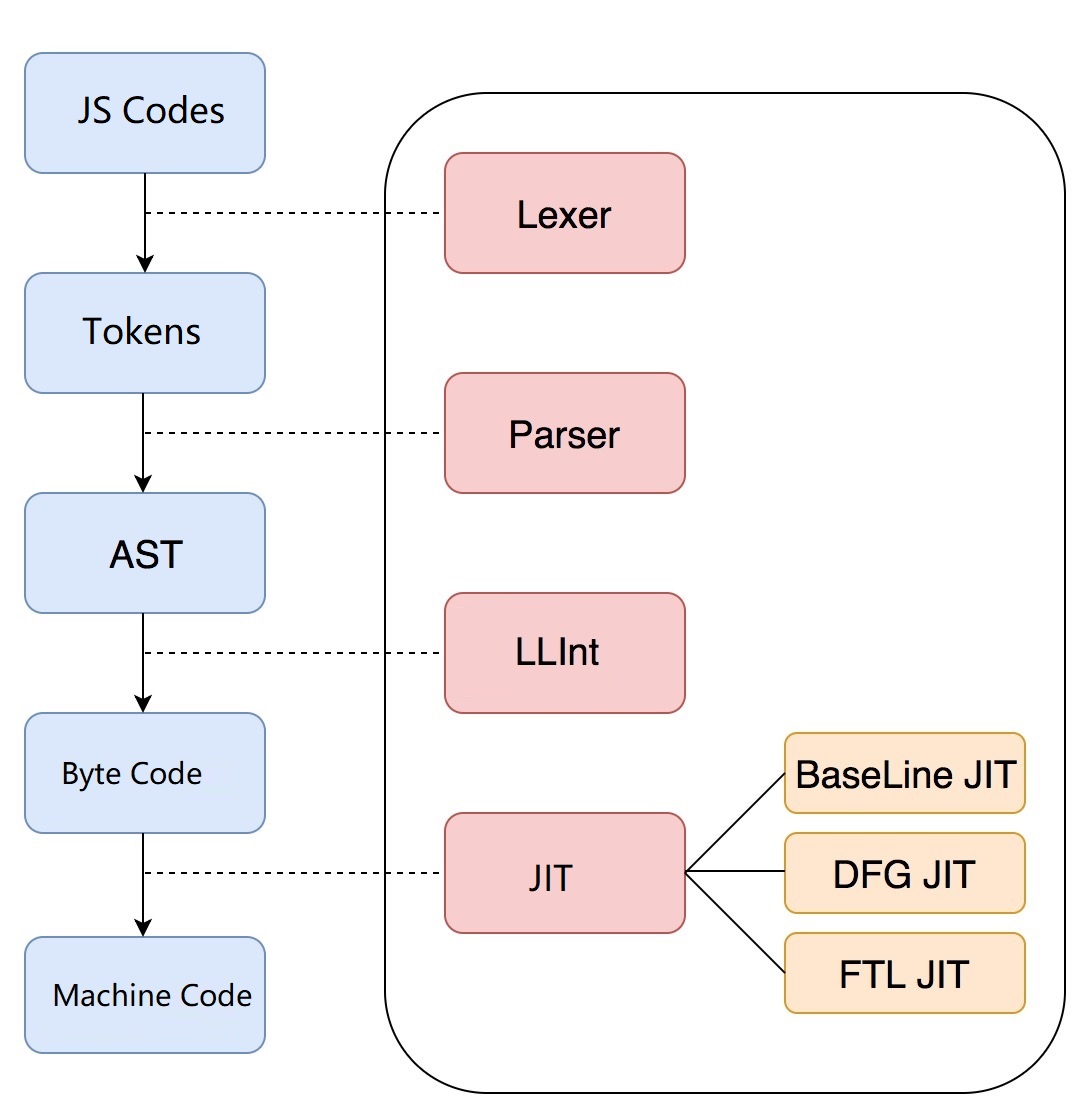
- Lexer: lexical analysis
The lexer will break down our source codes into a series of JS Tokens
1 | const a = 1; |
Some example tokens from above code snippet
Code | Token |
---|---|
const | Keyword |
a | Identifier |
= | EQUAL |
1 | Number |
; | SEMICOLON |
For more detail, we can directly check in source code:
https://github.com/WebKit/WebKit/blob/main/Source/JavaScriptCore/parser/Lexer.cpp
- Parser: syntax analysis
The parser will build an abstract syntax tree from above tokens. The AST represents code structural detail & meaning of each node.
Still for above sample codes, example AST would be like below:
1 | { |
Visualize Generator: https://esprima.org/demo/parse.html
LLInt: lower level interpreter
Translate above AST into ByteCodeJIT
Modern JS engine usually contains multiple tiers of JIT for best performance optimization, for JSCore:- Baseline JIT: as name, it directly compiles bytecode operation into native code instruction with specific template, no additional logic, no optimization.
- Data Flow Graph JIT: the DFG JIT has an intermediate representation (IR) which translate codes instruction into a data flow graph, and then perform complex optimizations base on codes context flow.
- Faster Than Light JIT
https://webkit.org/blog/3362/introducing-the-webkit-ftl-jit/
All above processes are running in single thread. To allow web app handling multi-thread tasks asynchronously, WebWorker & EventLoop are involved.
WebWorker is a separated OS level thread provided by browser core, communicate with main/UI thread with event driven mechanism, this communication mechanism is called EventLoop.
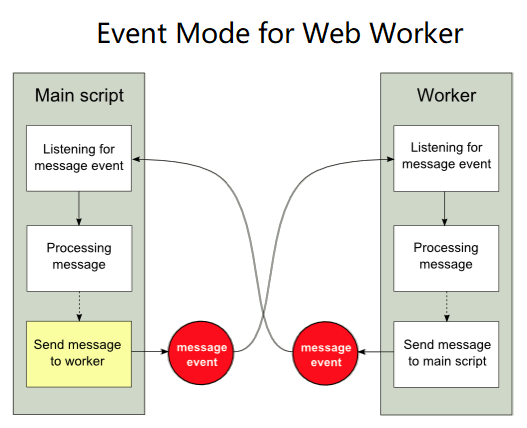
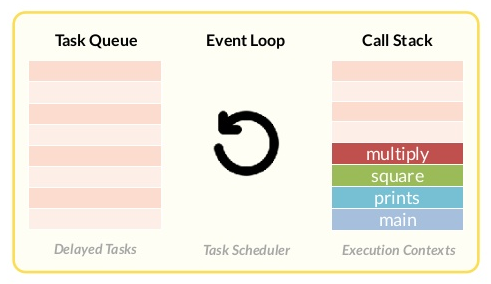
Pain Points of JavaScript
Javascript is rapid development / highly deliverable language, but was not designed to be executed with high performance, first version is designed within 10 days.
- Slow execution, CPU resource sensitive
- Single thread
- Fragmentation of ecosystem brings package quality/quantity issue
- Dynamic type not friendly for large-scale development
Cutting Edge Research
WebAssembly
- It’s a new standard by W3C, supported by mainstream modern web browsers.
- It’s a VM and compilation target from different languages: C/C++, Go/Rust, C#/Java/Swift…
- Compact binary format with near native speed.
- Shares same security policies in web browser sandbox.
- Goal is to handle high performance requirement, not to replace JS.
Get Start with WebAssemble: https://webassembly.org/getting-started/developers-guide/
WebAssembly Examples
- TensorFlow.js WASM backend to be 10-30x faster than the plain JS (CPU) backend across our models
- AutoCAD, GoogleEarth, Unity
- Windows 2000 in web browser
WebAssembly Ecosystem
- emscripten Compiler to WebAssembly for C/C++, convert OpenGL to WebGL.
- QT WebAssembly Build Qt application to webpage
- Go Official support in Golang.
- Yew Rust framework for creating multi-threaded front-end web apps with WebAssembly. Not production ready yet.
- Blazor WebAssembly
Not yet included in .net Core LTS. Discussion- Telerik
- DevExpress
- Ant Design Blazor
Performance Benchmark
When to consider WebAssembly ?
- High Performance Demand
- App Deployment Convenience
- Cross Platform Compilation Target
- Secure Sandbox Environment for Native Codes (Container service…)
- Tomorrow
WebGL
Render 2D/3D graphics on WebBrowser with GPU.
Makes GPU computing is possible for web apps.
e.g. https://blog.logrocket.com/ai-in-browsers-comparing-tensorflow-onnx-and-webdnn-for-image-classification/